Description
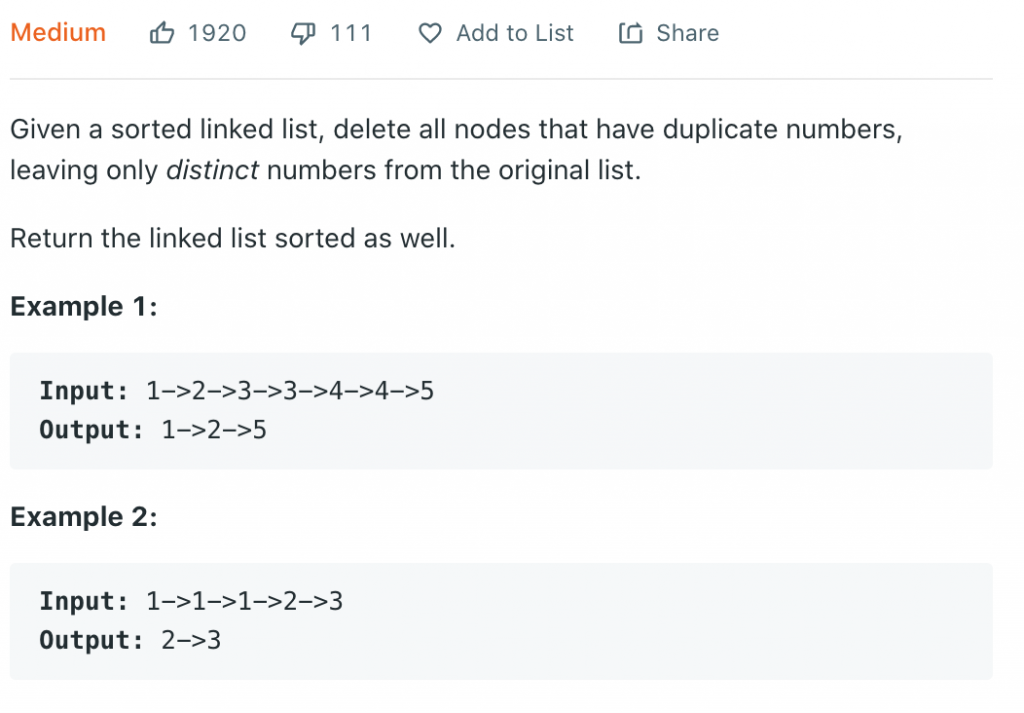
Submission
With fake head and nested iterative deletion, this should be faster. But I’ll just skip it.
/** * Definition for singly-linked list. * struct ListNode { * int val; * ListNode *next; * ListNode() : val(0), next(nullptr) {} * ListNode(int x) : val(x), next(nullptr) {} * ListNode(int x, ListNode *next) : val(x), next(next) {} * }; */ class Solution { public: ListNode* deleteDuplicates(ListNode* head) { if(!head || !head->next) return head; bool removeHead = false; if(head->val == head->next->val) removeHead = true; unordered_map<int, int> counter; for(ListNode* ptr = head; ptr != nullptr; ptr = ptr->next) { counter[ptr->val]++; } for(ListNode *ptr = head->next, *prevNode = head; ptr != nullptr; ptr = ptr->next) { auto iter = counter.find(ptr->val); if(iter->second > 1) { prevNode->next = ptr->next; } else { prevNode = ptr; } } // handle the head if(removeHead) { head = head->next; } return head; } };
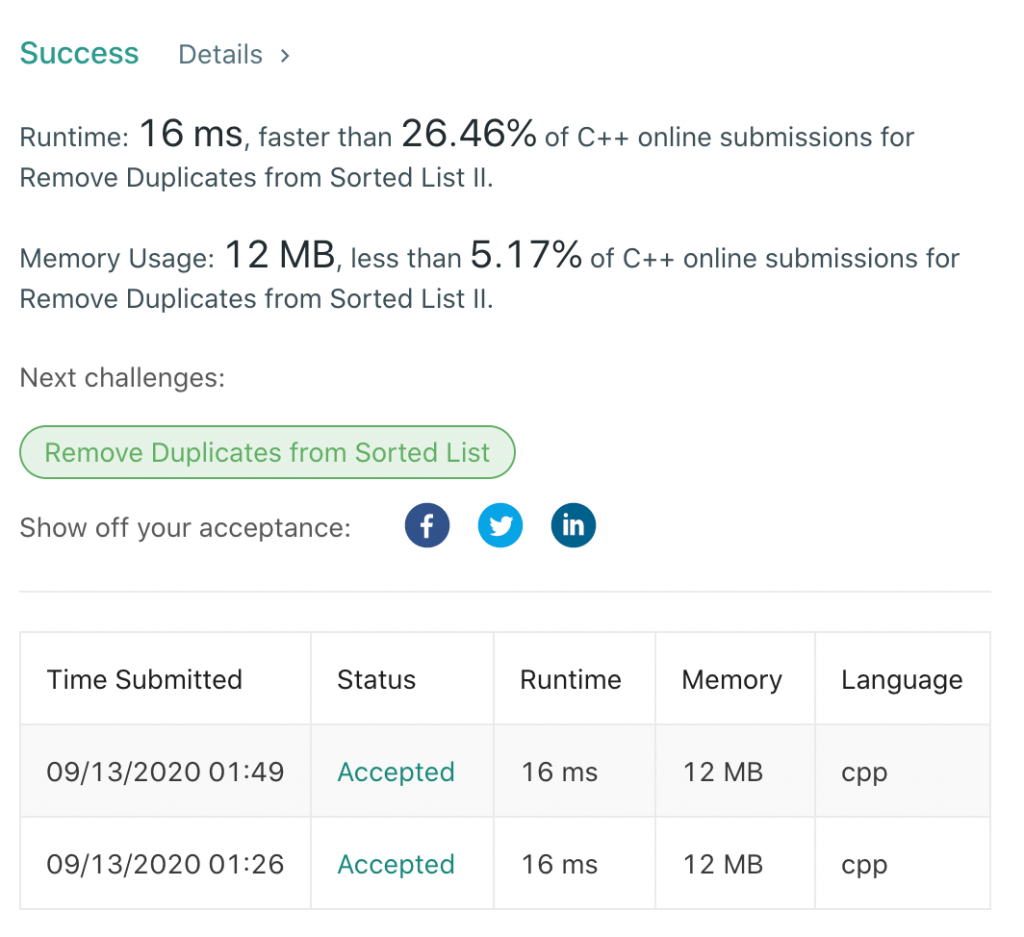
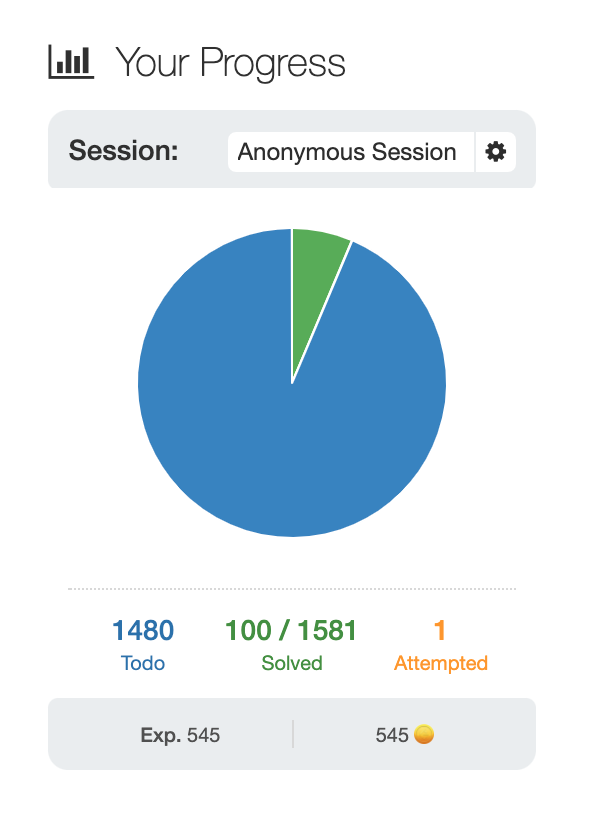