Description
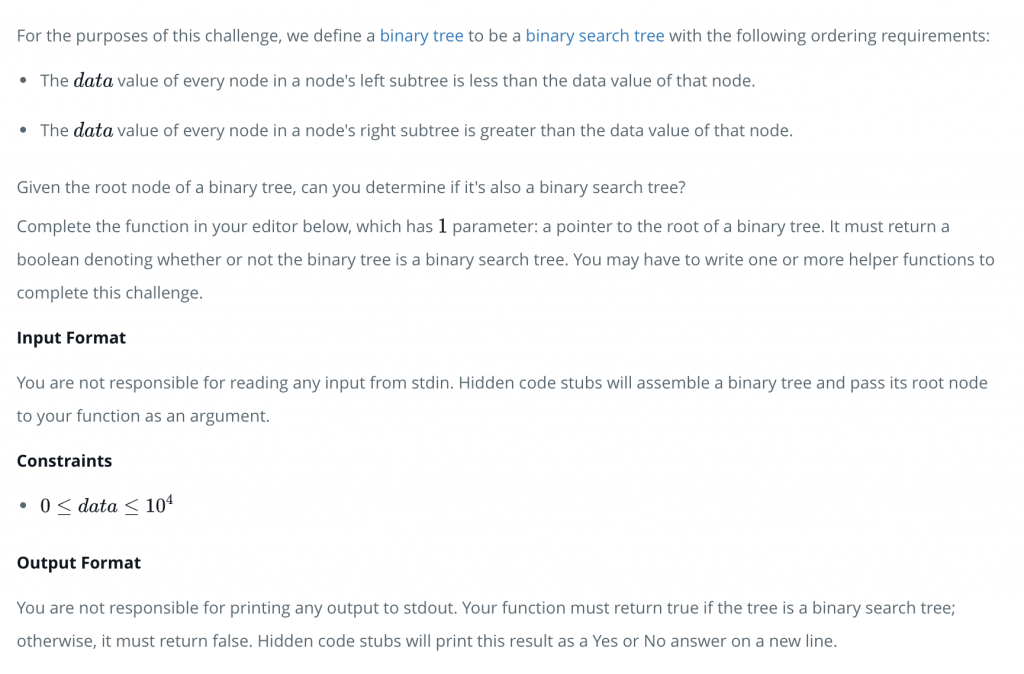
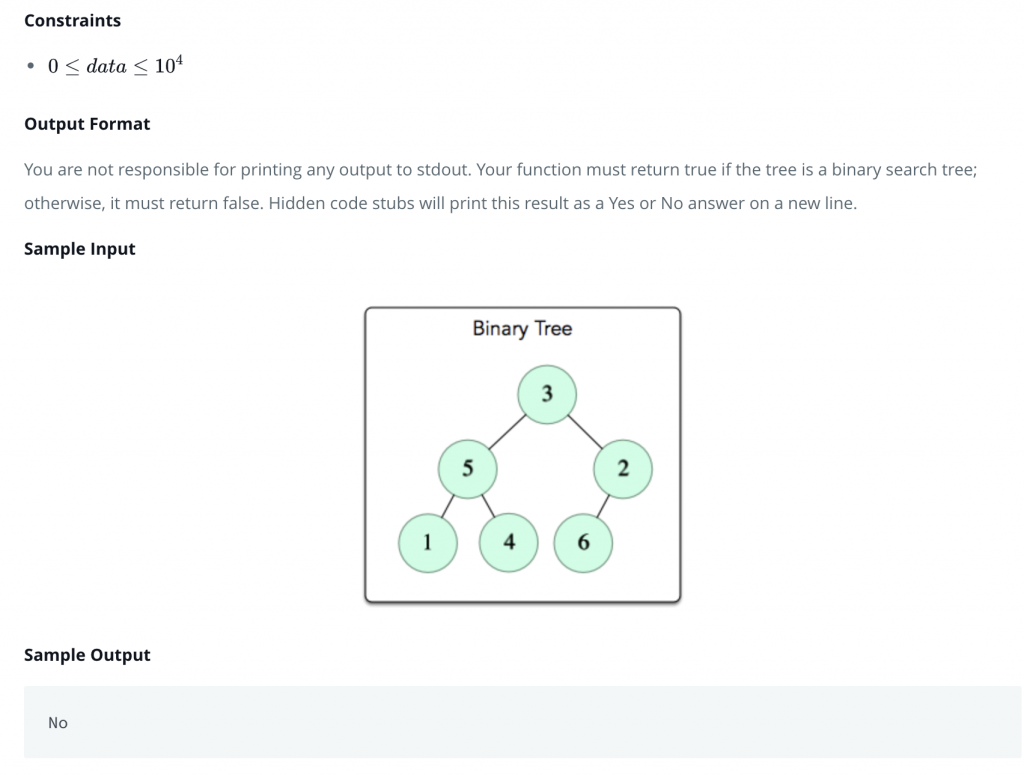
Submission
The special case should be considered
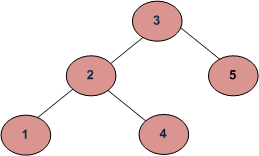
/* Hidden stub code will pass a root argument to the function below. Complete the function to solve the challenge. Hint: you may want to write one or more helper functions. The Node struct is defined as follows: struct Node { int data; Node* left; Node* right; } */ bool checkBSTUtil(Node* root, int minimum, int maximum) { if(root == NULL) return true; if(root->data < minimum || root->data > maximum) return false; return checkBSTUtil(root->left, minimum, root->data - 1) && checkBSTUtil(root->right, root->data + 1, maximum); } bool checkBST(Node* root) { return checkBSTUtil(root, -1, 20000); }