Description
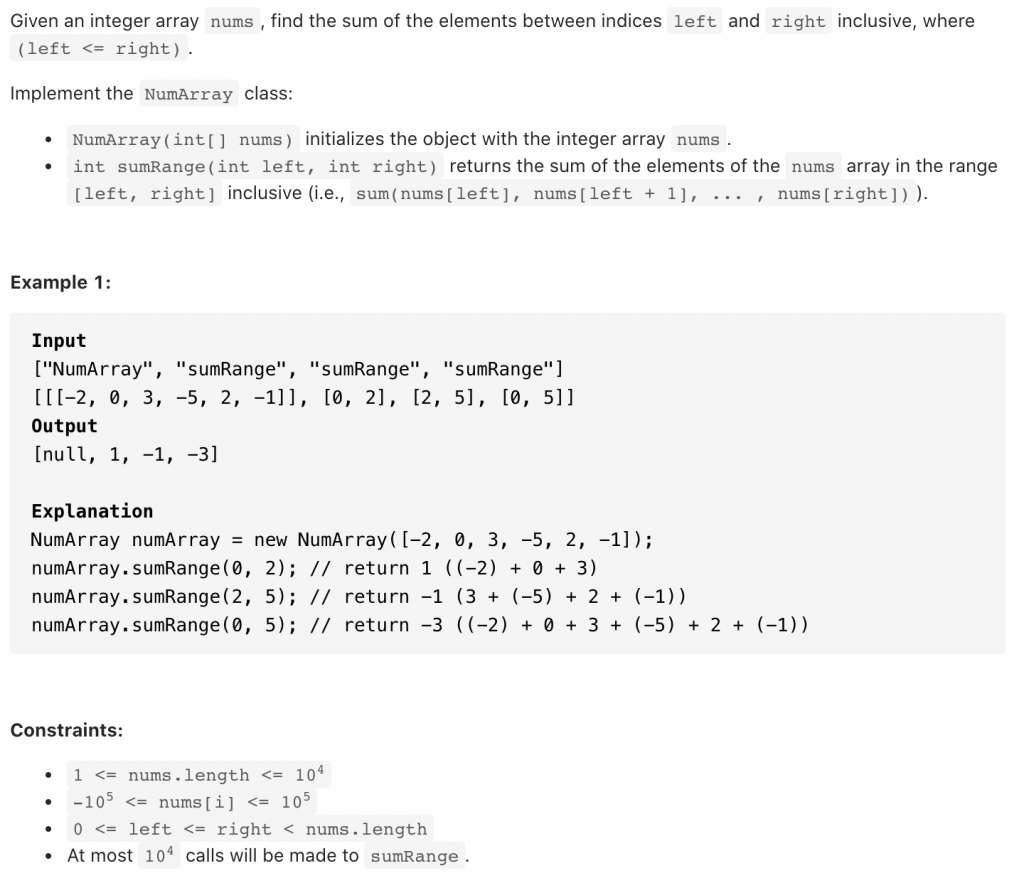
Submission
class NumArray { vector<int> presum; public: NumArray(vector<int>& nums) { nums.insert(nums.begin(), 0); int n = nums.size(); presum.resize(n); presum[0] = 0; for(int i = 1; i < n; ++i) { presum[i] = presum[i-1] + nums[i]; } } int sumRange(int left, int right) { return presum[right+1] - presum[left]; } }; /** * Your NumArray object will be instantiated and called as such: * NumArray* obj = new NumArray(nums); * int param_1 = obj->sumRange(left,right); */
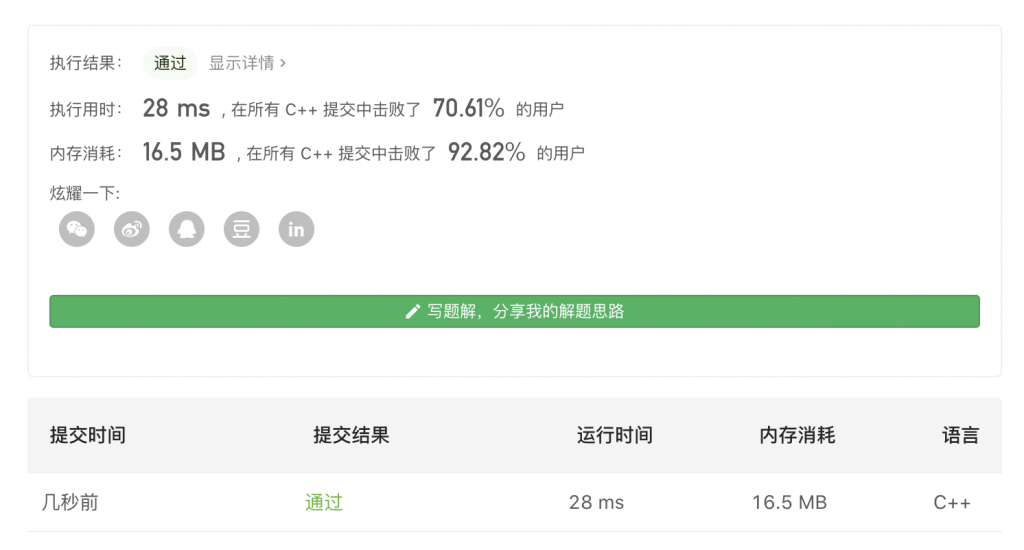