Built-in Data Types
Test Program
int main() { cout << "Size of char : " << sizeof(char) << " byte" << endl; cout << "Size of int : " << sizeof(int) << " bytes" << endl; cout << "Size of short int : " << sizeof(short int) << " bytes" << endl; cout << "Size of long int : " << sizeof(long int) << " bytes" << endl; cout << "Size of signed long int : " << sizeof(signed long int) << " bytes" << endl; cout << "Size of unsigned long int : " << sizeof(unsigned long int) << " bytes" << endl; cout << "Size of float : " << sizeof(float) << " bytes" <<endl; cout << "Size of double : " << sizeof(double) << " bytes" << endl; cout << "Size of wchar_t : " << sizeof(wchar_t) << " bytes" <<endl; return 0; }
Output:
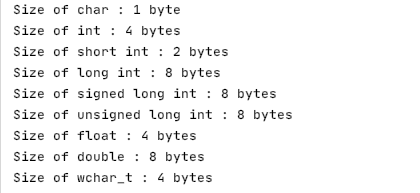
Struct
Note that there are paddings if a bigger data type is right before one with smaller size.
Test Program 1
int main() { struct A { // sizeof(int) = 4 int x; // Padding of 4 bytes // sizeof(double) = 8 double z; // sizeof(short int) = 2 short int y; // Padding of 6 bytes }; cout << "Size of struct: " << sizeof(struct A); return 0; }
Output:

Test Program 2
int main() { struct B { // sizeof(double) = 8 double z; // sizeof(int) = 4 int x; // sizeof(short int) = 2 short int y; // Padding of 2 bytes }; cout << "Size of struct: " << sizeof(struct B); return 0; }

Union
Union is as large as the largest member of it, including the padding size.
int main() { // "At least as big as the largest contained type", some paddings are added union { char all[13]; int foo; } record; printf("%d\n",sizeof(record.all)); printf("%d\n",sizeof(record.foo)); printf("%d\n",sizeof(record)); }
Output:
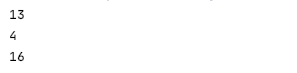
One Reply to “Size of C++ Data Types – Built-in, Structure and Union”