Description
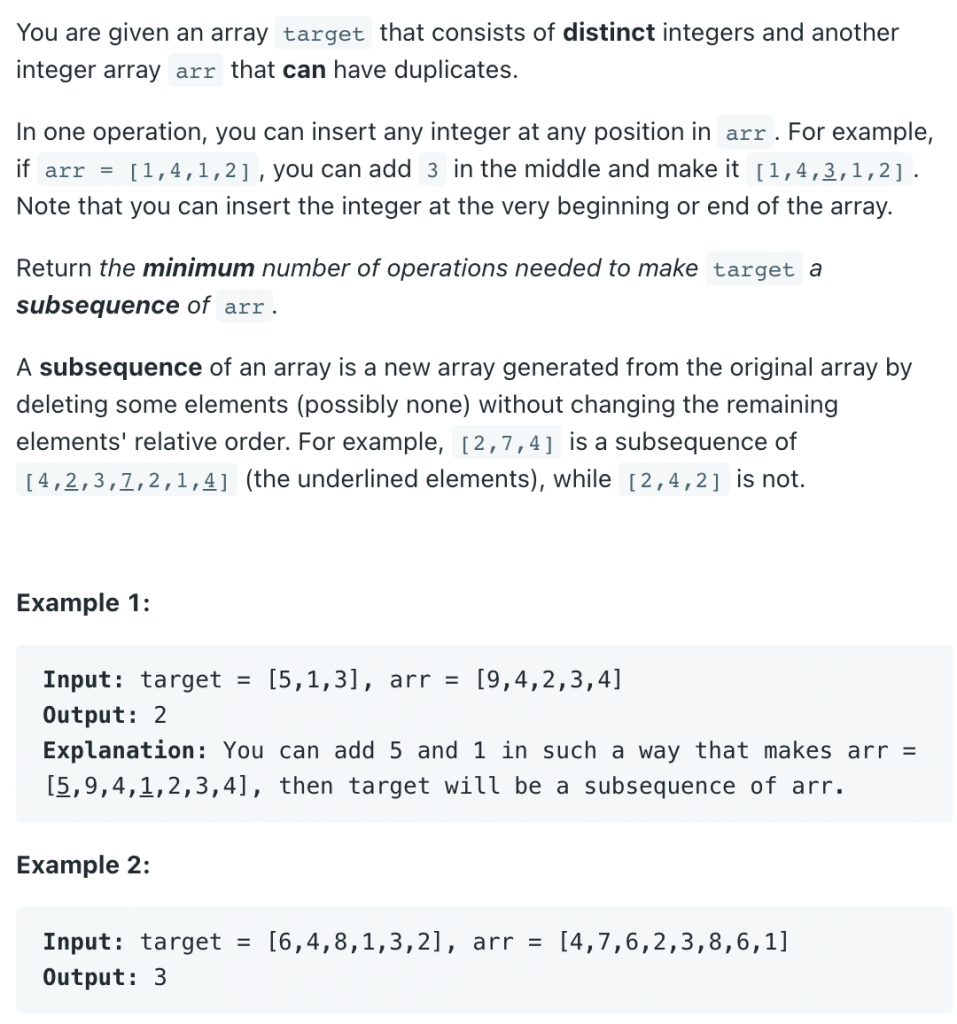
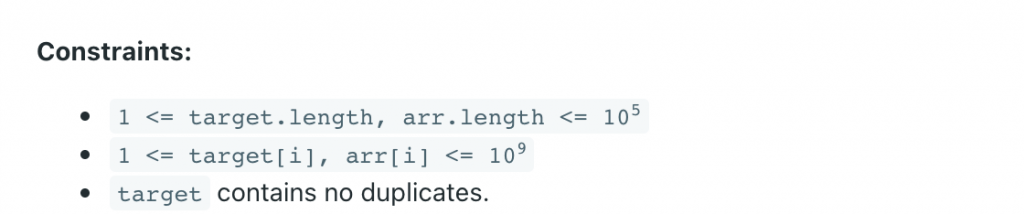
Submission
class Solution { public: int minOperations(vector<int>& target, vector<int>& arr) { // LIS map<int, int> Map; for(int i = 0; i < target.size(); ++i) { Map[target[i]] = i; } vector<int> q; for(auto x: arr) { if(Map.find(x) != Map.end()) { q.push_back(Map[x]); } } vector<int> s; for(int x : q) { if(s.empty() || x > s.back()) { s.push_back(x); } else { auto iter = lower_bound(s.begin(), s.end(), x); *iter = x; } } return target.size() - s.size(); } };
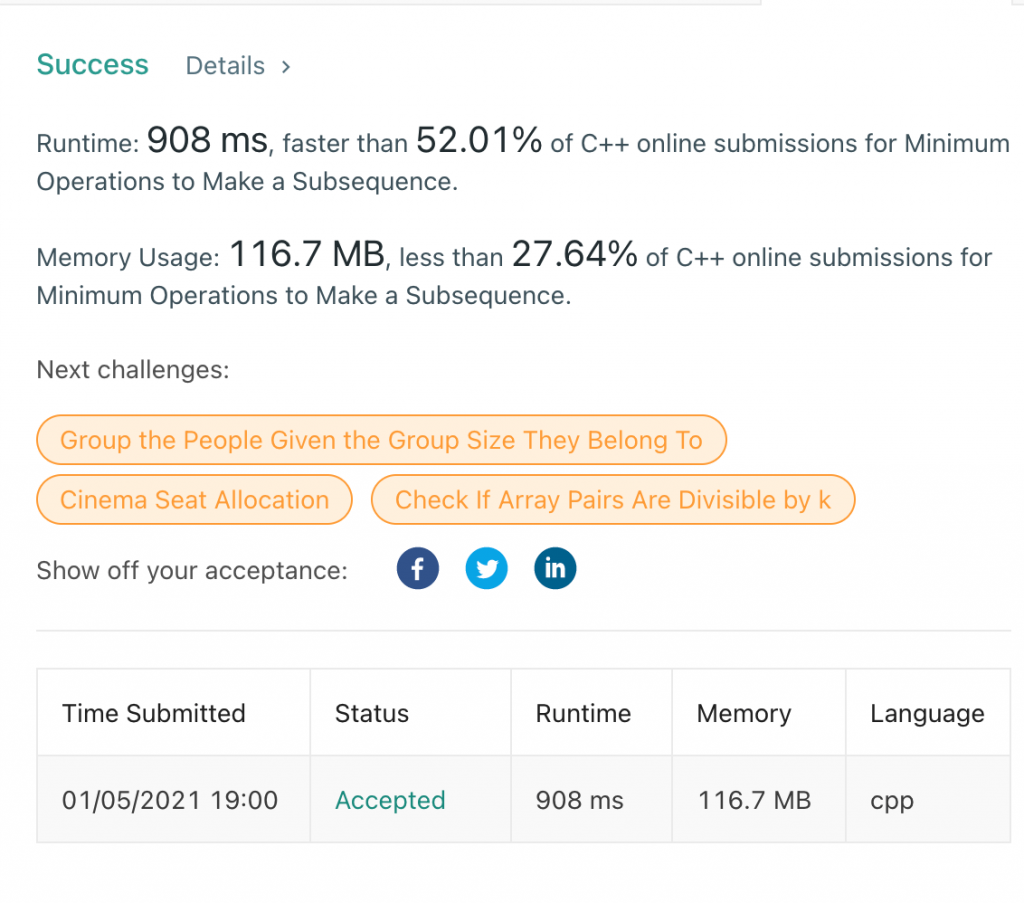