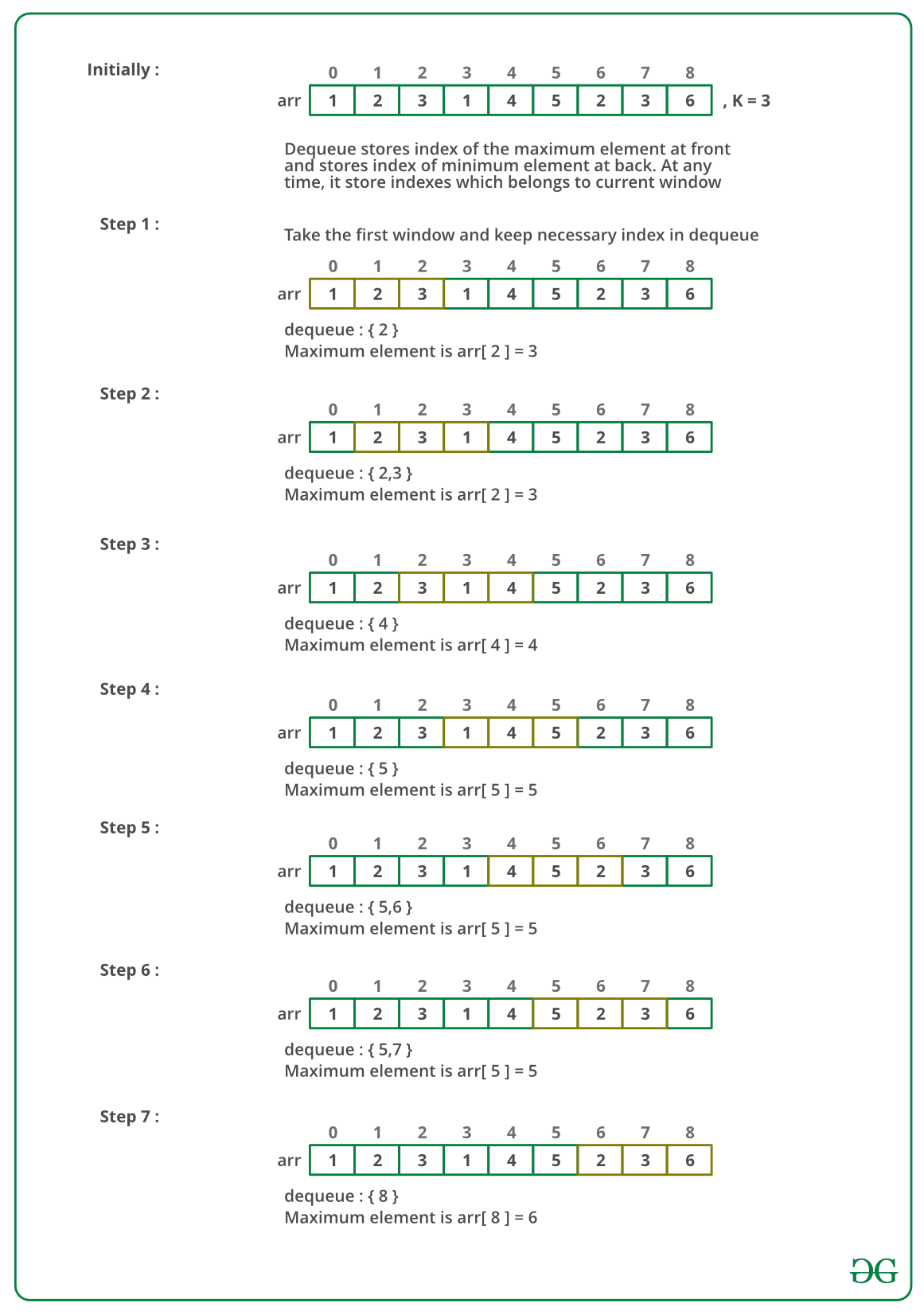
The crux here is that a number must be pushed into the deque on its turn since the bigger ones will be obsolete when they are out of the window. But for the previous ones, if they are smaller than the new one then they are useless since the new one can fully cover it.
#include <iostream> #include <deque> using namespace std; void printKMax(int arr[], int n, int k){ //Write your code here. deque<int> d; for ( int i = 0; i < k; i++ ) { while ( !d.empty() && arr[d.back()] <= arr[i] ) d.pop_back(); d.push_back(i); } for(int i = k; i < n; i++) { cout << arr[d.front()] << " "; // pop the ones out of the current windows size while(!d.empty() && d.front() <= i - k) { d.pop_front(); } while(!d.empty() && arr[d.back()] < arr[i]) { d.pop_back(); } d.push_back(i); } cout << arr[d.front()] << endl; } int main(){ int t; cin >> t; while(t>0) { int n,k; cin >> n >> k; int i; int arr[n]; for(i=0;i<n;i++) cin >> arr[i]; printKMax(arr, n, k); t--; } return 0; }