Description
Given a 32-bit signed integer, reverse digits of an integer.
Example 1:
Input: 123 Output: 321
Example 2:
Input: -123 Output: -321
Example 3:
Input: 120 Output: 21
Note:
Assume we are dealing with an environment, which could only store integers within the 32-bit signed integer range: [−231, 231 − 1]. For the purpose of this problem, assume that your function returns 0 when the reversed integer overflows.
Submission
class Solution { public int reverse(int x) { int sign = 1; if(x < 0) { sign = -1; x = -x; } String sX = Integer.toString(x).trim(); StringBuilder sb = new StringBuilder(); for(int i = sX.length() - 1; i >= 0; i--) { sb.append(sX.charAt(i)); } try { return sign * Integer.parseInt(sb.toString()); } catch (Exception e) { return 0; } } }
For small integers, it will work, but for larger ones, it won’t work if without the exception-handling part.
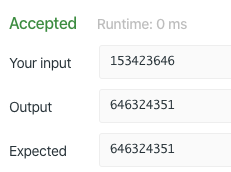
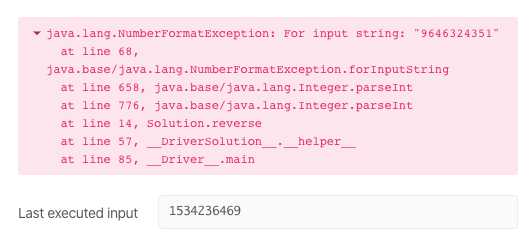
Solution
If we are to implement a library function, we should implement the function like the following.
class Solution { public int reverse(int x) { int rev = 0; while (x != 0) { int pop = x % 10; x /= 10; if (rev > Integer.MAX_VALUE/10 || (rev == Integer.MAX_VALUE / 10 && pop > 7)) return 0; if (rev < Integer.MIN_VALUE/10 || (rev == Integer.MIN_VALUE / 10 && pop < -8)) return 0; rev = rev * 10 + pop; } return rev; } }